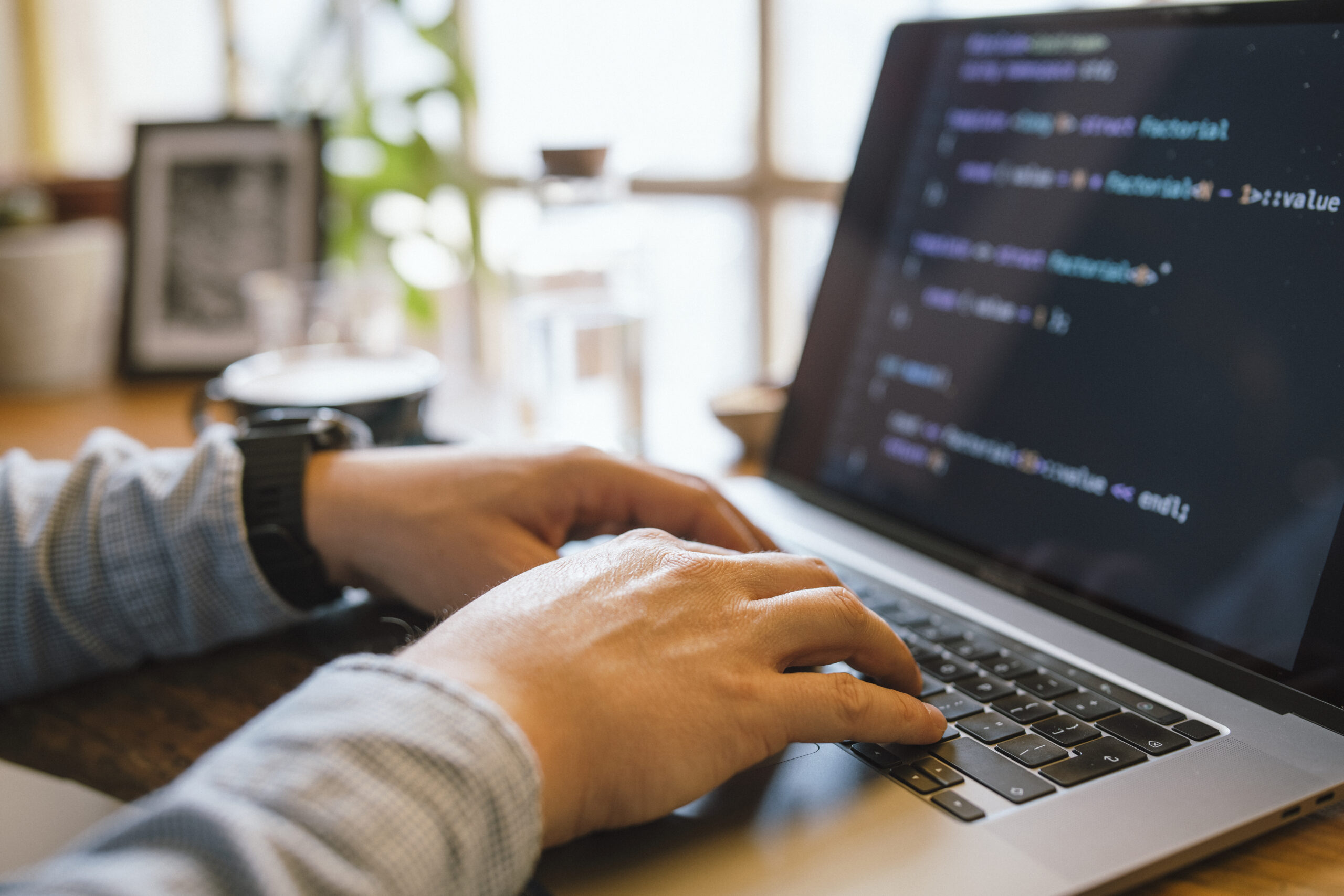
Debugging is one of the most vital — nonetheless frequently disregarded — techniques inside of a developer’s toolkit. It's not just about fixing broken code; it’s about comprehending how and why items go Mistaken, and Mastering to Assume methodically to unravel challenges successfully. Whether or not you're a beginner or a seasoned developer, sharpening your debugging abilities can save hours of frustration and significantly enhance your productivity. Here are quite a few procedures that will help builders degree up their debugging sport by me, Gustavo Woltmann.
Master Your Applications
On the list of fastest approaches developers can elevate their debugging skills is by mastering the applications they use on a daily basis. Even though creating code is a single A part of development, recognizing tips on how to communicate with it effectively all through execution is Similarly essential. Modern progress environments arrive Geared up with highly effective debugging capabilities — but lots of developers only scratch the surface of what these instruments can do.
Choose, by way of example, an Built-in Improvement Ecosystem (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These applications enable you to set breakpoints, inspect the worth of variables at runtime, phase through code line by line, and in many cases modify code within the fly. When made use of accurately, they let you observe accurately how your code behaves for the duration of execution, which is priceless for monitoring down elusive bugs.
Browser developer resources, for instance Chrome DevTools, are indispensable for front-conclude builders. They permit you to inspect the DOM, watch network requests, look at real-time efficiency metrics, and debug JavaScript during the browser. Mastering the console, sources, and network tabs can convert aggravating UI difficulties into workable tasks.
For backend or technique-amount developers, resources like GDB (GNU Debugger), Valgrind, or LLDB present deep control above jogging procedures and memory management. Understanding these instruments can have a steeper Studying curve but pays off when debugging efficiency difficulties, memory leaks, or segmentation faults.
Further than your IDE or debugger, turn out to be cozy with Model Regulate systems like Git to know code historical past, uncover the precise instant bugs were introduced, and isolate problematic adjustments.
Eventually, mastering your equipment suggests likely outside of default settings and shortcuts — it’s about creating an intimate understanding of your advancement surroundings to ensure when troubles occur, you’re not missing in the dead of night. The higher you recognize your instruments, the greater time it is possible to commit fixing the actual issue instead of fumbling through the process.
Reproduce the Problem
One of the most critical — and infrequently forgotten — techniques in powerful debugging is reproducing the trouble. Just before jumping into your code or building guesses, developers require to produce a reliable natural environment or situation exactly where the bug reliably seems. With no reproducibility, repairing a bug becomes a activity of possibility, usually leading to squandered time and fragile code adjustments.
The first step in reproducing a challenge is gathering as much context as is possible. Request questions like: What steps led to The difficulty? Which surroundings was it in — development, staging, or generation? Are there any logs, screenshots, or error messages? The greater detail you may have, the less difficult it becomes to isolate the precise conditions underneath which the bug occurs.
When you finally’ve collected plenty of info, seek to recreate the trouble in your local natural environment. This could indicate inputting the same knowledge, simulating similar consumer interactions, or mimicking system states. If The problem seems intermittently, think about producing automatic exams that replicate the sting conditions or state transitions included. These checks not just enable expose the problem but in addition reduce regressions in the future.
Often, The difficulty may be surroundings-precise — it'd occur only on specified functioning systems, browsers, or below distinct configurations. Working with tools like virtual devices, containerization (e.g., Docker), or cross-browser screening platforms is often instrumental in replicating such bugs.
Reproducing the trouble isn’t only a action — it’s a way of thinking. It requires patience, observation, along with a methodical strategy. But as soon as you can continually recreate the bug, you might be now midway to correcting it. With a reproducible scenario, You should use your debugging resources a lot more properly, take a look at probable fixes properly, and converse additional Plainly with the staff or people. It turns an summary grievance into a concrete challenge — and that’s where builders prosper.
Read through and Recognize the Error Messages
Mistake messages are sometimes the most useful clues a developer has when one thing goes Improper. Instead of seeing them as disheartening interruptions, builders need to find out to treat mistake messages as immediate communications from your method. They often show you what exactly occurred, where it transpired, and often even why it occurred — if you know the way to interpret them.
Get started by looking at the concept cautiously As well as in whole. A lot of developers, especially when less than time strain, glance at the 1st line and right away begin earning assumptions. But deeper during the error stack or logs may lie the genuine root result in. Don’t just duplicate and paste error messages into search engines — examine and realize them first.
Crack the mistake down into components. Can it be a syntax error, a runtime exception, or simply a logic error? Will it level to a selected file and line amount? What module or functionality induced it? These thoughts can information your investigation and point you toward the liable code.
It’s also useful to be familiar with the terminology from the programming language or framework you’re working with. Error messages in languages like Python, JavaScript, or Java usually observe predictable patterns, and Finding out to acknowledge these can significantly accelerate your debugging system.
Some mistakes are obscure or generic, As well as in those circumstances, it’s important to look at the context where the mistake occurred. Examine linked log entries, enter values, and recent alterations while in the codebase.
Don’t ignore compiler or linter warnings either. These frequently precede more substantial challenges and supply hints about possible bugs.
In the end, error messages are certainly not your enemies—they’re your guides. Discovering to interpret them correctly turns chaos into clarity, assisting you pinpoint concerns more rapidly, lower debugging time, and turn into a extra economical and confident developer.
Use Logging Wisely
Logging is Probably the most potent resources within a developer’s debugging toolkit. When utilised properly, it offers true-time insights into how an application behaves, aiding you recognize what’s taking place beneath the hood with no need to pause execution or stage with the code line by line.
An excellent logging method begins with understanding what to log and at what level. Common logging concentrations involve DEBUG, Facts, Alert, ERROR, and FATAL. Use DEBUG for detailed diagnostic information and facts all through progress, Data for basic occasions (like effective start-ups), Alert for likely concerns that don’t break the applying, ERROR for actual complications, and Lethal once the method can’t go on.
Prevent flooding your logs with extreme or irrelevant information. Far too much logging can obscure significant messages and slow down your system. Deal with critical activities, point out alterations, input/output values, and significant selection details with your code.
Format your log messages Plainly and regularly. Contain context, such as timestamps, request IDs, and performance names, so it’s simpler to trace challenges in distributed units or multi-threaded environments. Structured logging (e.g., JSON logs) might make it even easier to parse and filter logs programmatically.
For the duration of debugging, logs let you observe how variables evolve, what conditions are fulfilled, and what branches of logic are executed—all without halting the program. They’re Primarily useful in output environments in which stepping as a result of code isn’t achievable.
On top of that, use logging frameworks and resources (like Log4j, Winston, or Python’s logging module) that guidance log rotation, filtering, and integration with checking dashboards.
In the end, intelligent logging is about balance and clarity. By using a well-believed-out logging tactic, you can decrease the time it will require to identify problems, achieve further visibility into your applications, and improve the Total maintainability and trustworthiness of your code.
Believe Just like a Detective
Debugging is not simply a technological task—it's a kind of investigation. To proficiently detect and repair bugs, developers need to tactic the procedure similar to a detective solving a mystery. This state of mind aids break down intricate difficulties into workable pieces and follow clues logically to uncover the root result in.
Commence by collecting evidence. Consider the indicators of the situation: mistake messages, incorrect output, or effectiveness difficulties. Identical to a detective surveys against the law scene, accumulate just as much suitable facts as you may devoid of leaping to conclusions. Use logs, take a look at situations, and consumer studies to piece with each other a clear picture of what’s going on.
Future, sort hypotheses. Check with by yourself: What may be leading to this conduct? Have any changes recently been built to the codebase? Has this issue happened ahead of beneath comparable circumstances? The intention should be to narrow down possibilities and establish likely culprits.
Then, check your theories systematically. Attempt to recreate the issue inside of a managed setting. Should you suspect a specific purpose or element, isolate it and verify if The difficulty persists. Just like a detective conducting interviews, ask your code thoughts and Permit click here the outcomes guide you closer to the truth.
Fork out near attention to smaller information. Bugs usually disguise while in the least predicted places—just like a missing semicolon, an off-by-just one error, or maybe a race situation. Be extensive and affected person, resisting the urge to patch The difficulty with out absolutely comprehension it. Temporary fixes could disguise the true trouble, only for it to resurface later on.
Last of all, preserve notes on Anything you attempted and figured out. Equally as detectives log their investigations, documenting your debugging method can help save time for future troubles and assistance Other people recognize your reasoning.
By thinking like a detective, developers can sharpen their analytical techniques, approach issues methodically, and turn into more practical at uncovering concealed problems in intricate devices.
Write Tests
Composing assessments is among the simplest ways to enhance your debugging expertise and Total progress performance. Checks not only assist catch bugs early and also function a security Internet that provides you self esteem when building variations towards your codebase. A well-tested software is much easier to debug mainly because it allows you to pinpoint exactly where and when a challenge happens.
Begin with unit exams, which give attention to personal functions or modules. These little, isolated tests can quickly expose whether a selected bit of logic is Performing as predicted. Each time a check fails, you instantly know exactly where to look, noticeably lessening enough time put in debugging. Unit checks are Primarily handy for catching regression bugs—troubles that reappear right after previously remaining fastened.
Upcoming, integrate integration tests and close-to-conclusion exams into your workflow. These help make sure several areas of your software get the job done with each other smoothly. They’re specially beneficial for catching bugs that occur in advanced techniques with multiple parts or solutions interacting. If a little something breaks, your exams can tell you which Section of the pipeline failed and underneath what circumstances.
Producing exams also forces you to definitely Feel critically regarding your code. To test a element effectively, you would like to comprehend its inputs, envisioned outputs, and edge circumstances. This volume of knowing The natural way qualified prospects to raised code structure and less bugs.
When debugging a difficulty, creating a failing examination that reproduces the bug can be a powerful initial step. As soon as the check fails continually, you are able to center on correcting the bug and view your examination go when the issue is settled. This technique makes certain that exactly the same bug doesn’t return Sooner or later.
To put it briefly, writing exams turns debugging from a discouraging guessing activity into a structured and predictable method—supporting you capture extra bugs, quicker and even more reliably.
Just take Breaks
When debugging a tough difficulty, it’s easy to become immersed in the trouble—watching your display screen for hrs, hoping Alternative after Answer. But Just about the most underrated debugging equipment is just stepping away. Using breaks aids you reset your brain, lessen stress, and sometimes see The problem from a new viewpoint.
When you are far too near to the code for way too prolonged, cognitive tiredness sets in. You could possibly start off overlooking clear glitches or misreading code that you just wrote just hrs previously. Within this state, your Mind will become a lot less successful at dilemma-fixing. A short wander, a espresso break, or perhaps switching to a different endeavor for ten–15 minutes can refresh your concentrate. Many builders report acquiring the basis of an issue when they've taken time and energy to disconnect, allowing their subconscious function during the qualifications.
Breaks also support stop burnout, especially through more time debugging sessions. Sitting down in front of a monitor, mentally caught, is not just unproductive but will also draining. Stepping away enables you to return with renewed Electrical power plus a clearer state of mind. You may perhaps out of the blue discover a lacking semicolon, a logic flaw, or even a misplaced variable that eluded you before.
In case you’re stuck, a fantastic rule of thumb will be to set a timer—debug actively for forty five–60 minutes, then take a five–10 moment break. Use that point to maneuver close to, extend, or do one thing unrelated to code. It may well truly feel counterintuitive, Primarily below limited deadlines, however it essentially leads to more rapidly and more effective debugging Over time.
To put it briefly, taking breaks will not be an indication of weakness—it’s a wise system. It gives your brain Place to breathe, improves your viewpoint, and can help you steer clear of the tunnel vision That usually blocks your development. Debugging is a mental puzzle, and rest is a component of resolving it.
Learn From Each and every Bug
Just about every bug you encounter is more than just A brief setback—It is really an opportunity to expand being a developer. Irrespective of whether it’s a syntax error, a logic flaw, or perhaps a deep architectural concern, each can train you a little something valuable should you make time to replicate and assess what went Completely wrong.
Start by asking your self several essential inquiries when the bug is solved: What brought about it? Why did it go unnoticed? Could it have been caught earlier with better practices like unit testing, code reviews, or logging? The answers often expose blind places in the workflow or being familiar with and assist you Establish much better coding behaviors transferring ahead.
Documenting bugs can be a superb routine. Preserve a developer journal or preserve a log where you Take note down bugs you’ve encountered, the way you solved them, and That which you acquired. Eventually, you’ll begin to see designs—recurring problems or common issues—you can proactively keep away from.
In crew environments, sharing Everything you've learned from the bug using your peers is usually In particular effective. Whether or not it’s via a Slack concept, a short generate-up, or a quick understanding-sharing session, encouraging Other folks avoid the exact situation boosts group performance and cultivates a more powerful learning lifestyle.
Much more importantly, viewing bugs as classes shifts your attitude from frustration to curiosity. In place of dreading bugs, you’ll begin appreciating them as critical areas of your development journey. All things considered, a few of the finest developers are certainly not the ones who publish perfect code, but individuals who continuously understand from their errors.
In the long run, Every bug you correct provides a fresh layer towards your skill set. So upcoming time you squash a bug, take a second to replicate—you’ll occur away a smarter, far more able developer due to it.
Summary
Improving upon your debugging abilities normally takes time, observe, and patience — even so the payoff is large. It makes you a more productive, self-assured, and able developer. The next time you are knee-deep in the mysterious bug, try to remember: debugging isn’t a chore — it’s an opportunity to become far better at That which you do.